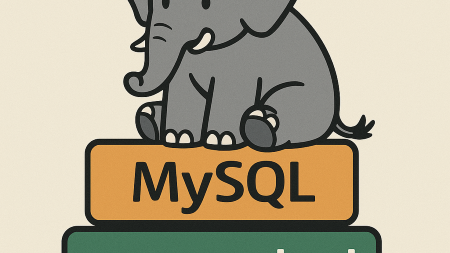
Before integrating Memcached with PHP, ensure it is installed and running on your server.
Installing Memcached:
sudo apt update
sudo apt install memcached libmemcached-tools
sudo yum install memcached
sudo systemctl start memcached
sudo systemctl enable memcached
sudo apt install php-memcached
sudo systemctl restart php-fpm
sudo systemctl restart apache2
You can also have multiple versions of php so when you restart it can be php8.3-fpm.
Step 1: Connect to Memcached in PHP
Use the Memcached class to connect to Memcached.
<?php
$memcached = new Memcached();
$memcached->addServer('127.0.0.1', 11211); // Replace with your Memcached server details
Step 2: Store and Retrieve Data
$key = 'user_data_1';
$data = ['name' => 'John Doe', 'email' => 'john@example.com'];
$memcached->set($key, $data, 300); // Cache for 300 seconds
$cachedData = $memcached->get($key);
if ($cachedData) {
echo 'Data from cache:';
print_r($cachedData);
} else {
echo 'No cache found!';
}
Step 3: Cache SQL Query Results
$key = 'query_result_users';
$result = $memcached->get($key);
if (!$result) {
// If not cached, fetch data from the database
$pdo = new PDO('mysql:host=localhost;dbname=testdb', 'username', 'password');
$stmt = $pdo->query('SELECT * FROM users');
$result = $stmt->fetchAll(PDO::FETCH_ASSOC);
// Cache the result
$memcached->set($key, $result, 300);
}
// Use the cached or fetched data
print_r($result);
You can invalidate cache data by using:
$memcached->delete('query_result_users'); //or the key you set up
Integrating PHP and SQL with Memcached is a straightforward way to enhance the performance of your web applications. By caching frequently accessed data, you can significantly reduce database load, improve response times, and create a smoother user experience. With the steps and best practices outlined above, you’re ready to optimize your application’s performance with Memcached.
Technical writer and developer at DigitalCodeLabs with expertise in web development and server management.
Get the latest posts delivered straight to your inbox.